Blink rate
In this example, you will use a potentiometer to control the flashing speed of an LED light. It will change as you turn it.
What you need​
- SwiftIO Micro (or SwiftIO board)
- Breadboard
- Potentiometer
- LED
- 330ohm resistor
- Jumper wires
Circuit​
- Place the potentiometer onto the breadboard. Connect the legs on the left to pin 3V3, connect the second leg to pin A0, and the third leg to pin GND.
- Place the LED onto the breadboard. The long leg of LED goes to D1 through a resistor. The short leg connects to GND.
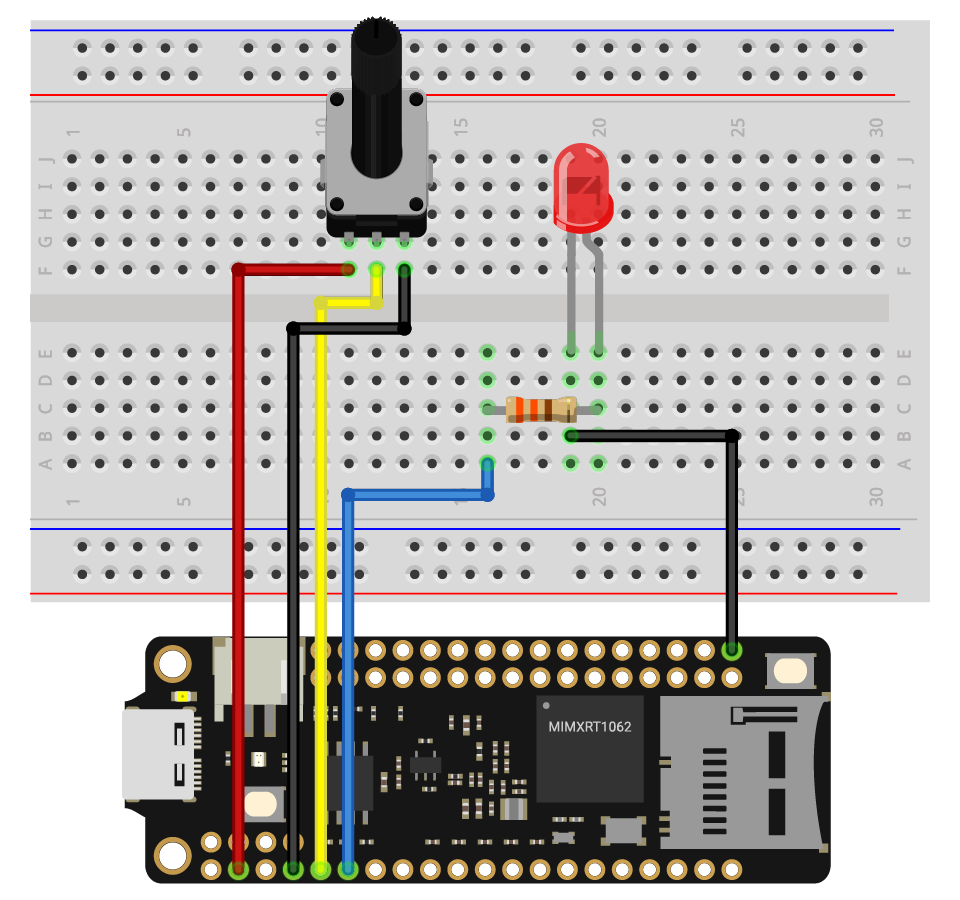
Example code​
Open the project BlinkRate
in the folder MadExamples
/Examples
/SimpleIO
if you downloaded the examples.
// Read the analog input and use it to set the rate of LED blink.
// Import the library to enable the relevant classes and functions.
import SwiftIO
import MadBoard
@main
public struct BlinkRate {
public static func main() {
// Initialize an analog input and a digital output pin that the components are connected to.
let sensor = AnalogIn(Id.A0)
let led = DigitalOut(Id.D1)
// Enable the LED to blink over and over again.
while true {
// Read the input voltage in percentage.
let value = sensor.readRawValue()
// Change the current LED state.
led.toggle()
// Keep the LED on or off for a certain period determined by the value you get.
sleep(ms: value)
}
}
}
Code analysis​
let value = sensor.readRawValue()
The method .readRawValue()
reads the current raw value from the specified analog pin. Since the analog-to-digital converter on the Board has a resolution of 12-bit, the corresponding value would be 0-4095.
led.toggle()
The method .toggle()
, as the name suggests, changes the output level. If it's high level now, it will be changed to low, and vice versa.
sleep(ms: value)
The function sleep()
keeps the current state of the LED for a specified time decided by the value
. It makes sure that the time between each toggle is under control and changes with the potentiometer.
Reference​
DigitalOut - set whether the pin outputs a high or low voltage.
AnalogIn - read the voltage from an analog pin.
MadBoard - find the corresponding pin id of your board.