LED switch
In this example, you will use a pushbutton to control the LED. When you press the button, the LED turns on. When you release it, the LED turns off. The input signal will change as you press the button. Thus, you can set LED states according to input states.
What you need​
- SwiftIO Micro (or SwiftIO board)
- Breadboard
- Button
- Jumper wires
Circuit​
- Plug the button into the breadboard.
- Connect one leg on one side to the pin 3V3.
- Connect one leg on the other side to pin D0.
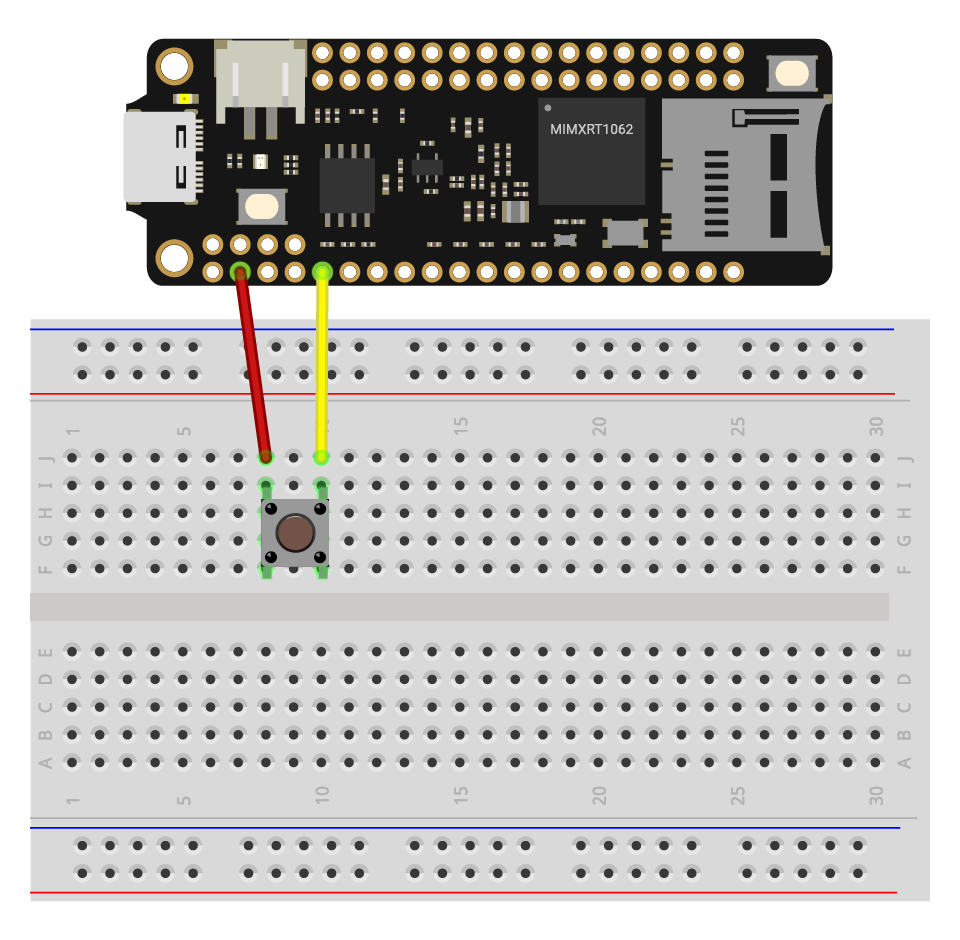
Example code​
Open the project LEDSwitch
in the folder MadExamples
/Examples
/SimpleIO
if you downloaded the examples.
// Read the input signal controlled by a button to turn on and off the LED.
// Import the library to enable the relevant classes and functions.
import SwiftIO
import MadBoard
@main
public struct LEDSwitch {
public static func main() {
// Initialize the red onboard LED.
let red = DigitalOut(Id.RED)
// Initialize a digital input pin D0 that the button is connected to.
let button = DigitalIn(Id.D0)
// Allow the button to control the LED all the time.
while true {
// Check the state of button. If it is pressed, the value will be true and then turn off the LED.
// Modify the code according to your button if necessary.
if button.read() {
red.write(false)
} else {
red.write(true)
}
}
}
}
Code analysis​
if button.read() {
red.write(false)
} else {
red.write(true)
}
First, you'll need to check the button state. The method .read()
reads the value from the digital input pin. The return value is a boolean value, either true
(1) or false
(0).
Then use if-else statement to decide the LED state according to the value. If the value is true
, the button has been pressed, so turn on the LED. Otherwise, turn off the LED.
If you have experience with Arduino, you may notice there's no pull-down resistor on the button. That's because our boards already have built-in pull resistors. Reference the Mode
in DigitalIn
class for more information.
Reference​
DigitalOut - set whether the pin outputs a high or low voltage.
DigitalIn - read the input value from a digital pin.
MadBoard - find the corresponding pin id of your board.